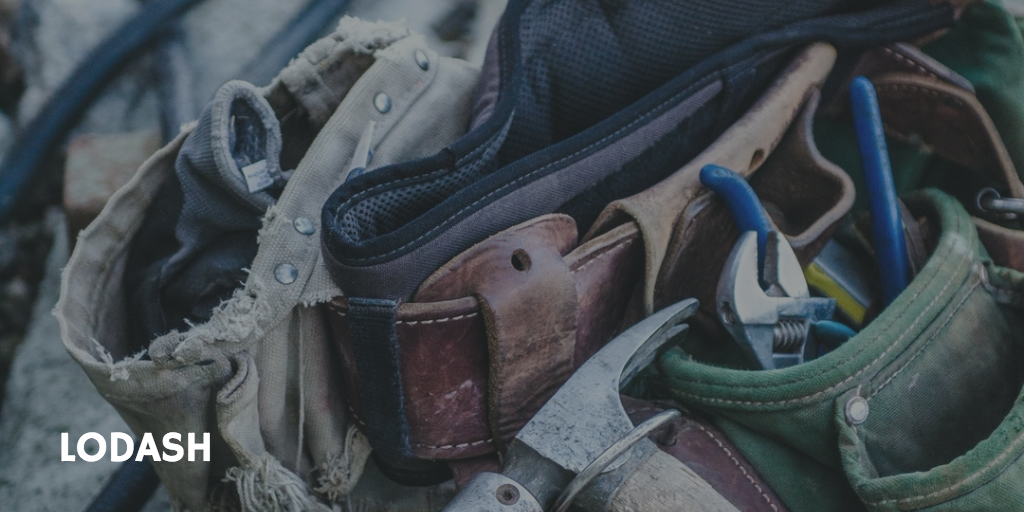
While writing your javascript applications you might find yourself implementing the same patterns over and over to work with arrays and objects. Some of these patterns you commonly encounter include looping over data to manipulate each item, filtering and sorting data based on specific criteria, and finding differences and commonality between multiple sets of data.
Instead of writing these workflows from scratch you might want to try a utility library such as lodash. Lodash is a modern javascript utility library that provides excellent features for working with arrays, objects, and collections. Here is a list of the common functions I will be covering in this article. The version of lodash used in this article is 4.5.1.
Common Lodash Functions:
_.forEach
_.map
_.uniq
_.difference
_.intersection
_.sortBy
_.filter
_.includes
_.some
Installation
You can download the source here or alternatively use npm or bower to install.
bower install lodash
OR
npm install lodash
You can require lodash in your node.js code or include the script in your client side javascript.
// Node.js
var _ = require('lodash');
OR
// Client side
// Similar to jQuery being exposed as $
// lodash will be exposed as _
<script src="path/to/lodash.js"></script>
1) _.forEach
This function will iterate over an array of items one at a time and let you perform whatever logic you need to. In this first example I'm going to loop over a collection of user objects combining their first and last names to make a new property called fullName.
var users = [
{
first: 'Keith',
last: 'Keough',
email: '[email protected]',
age: 35
},
{
first: 'Cassandra',
last: 'Wilcox',
email: '[email protected]',
age: 25
},
{
first: 'Ian',
last: 'Grail',
email: '[email protected]',
age: 21
},
{
first: 'Georgette',
last: 'Keough',
email: '[email protected]',
age: 25
}
];
Now that we have our user objects we will use forEach to loop over them. forEach accepts two arguments. The first argument is the array we are looping over. The second argument is a iteratee function where the first parameter is the individual item reference and the second is the current index of the iteration.
_.forEach(users, function(user, index) {
user.fullName = user.first + ' ' + user.last;
});
// Output
[{
age: 35,
email: "[email protected]",
first: "Keith",
fullName: "Keith Keough",
last: "Keough"
}, {
age: 25,
email: "[email protected]",
first: "Cassandra",
fullName: "Cassandra Wilcox",
last: "Wilcox"
}, {
age: 21,
email: "[email protected]",
first: "Ian",
fullName: "Ian Grail",
last: "Grail"
}, {
age: 25,
email: "[email protected]",
first: "Georgette",
fullName: "Georgette Keough",
last: "Keough"
}]
You can see that our output now has an additional property on each user object called fullName that has combined the users first and last together.
2) _.map
Like forEach, map will iterate over an array of values. However the map function returns a new array of values specified by the iteratee function.
Here we are using the same set of users from our forEach example. We can use map to create a new variable that will be a flattened array of all the users full names
var usersNames = _.map(users, function(user) {
return user.fullName;
});
// Output
["Keith Keough", "Cassandra Wilcox", "Ian Grail", "Georgette Keough"]
When you perform a map operation that is simply "plucking" off values from each of the collection items you can use the following shorthand to write less code.
var usersEmails = _.map(users, 'email');
// Output
["[email protected]", "[email protected]", "[email protected]", "[email protected]"]
Now lets use the map function to manipulate a dataset by performing some logical operation on it before we return the value to the new array.
var numbers = [5, 10, 50, 100];
var dividedByFive = _.map(numbers, function(number) {
return number / 5;
});
// Output
[1, 2, 10, 20]
3) _.uniq
The uniq function will take an array as an argument and return all unique values. In other words get rid of duplicates.
var numbers = [1, 5, 8, 10, 1, 5, 15, 42, 5, 56];
numbers = _.uniq(numbers);
// Output
[1, 5, 8, 10, 15, 42, 56]
4) _.difference
The difference function will return a new array of values that was different from the first array to the second array. It's important to note that the positions of the arguments will change the results.
var a = ['Keith', 'Ian', 'Georgette'];
var b = ['Cassie', 'Duke', 'Keith', 'Ian'];
var differenceA = _.difference(a, b);
var differenceB = _.difference(b, a);
// Output of differenceA
["Georgette"]
// Output of differenceB
["Cassie", "Duke"]
5) _.intersection
The intersection function will return a new array of the common values from the first array and the second array.
var a = ['Keith', 'Ian', 'Georgette'];
var b = ['Cassie', 'Duke', 'Keith', 'Ian'];
var intersection = _.intersection(a, b);
// Output
["Keith", "Ian"]
Now lets combine the intersection and difference functions to find the values that were added and removed from the first array to the second array.
var before = [1, 6, 8, 10];
var after = [1, 5, 8, 11];
var common = _.intersection(before, after);
// Output
[1, 8]
var newValues = _.difference(after, common);
// Output
[5, 11]
var removedValues = _.difference(before, common);
// Output
[6, 10]
6) _.sortBy
The sort by method allows you to sort arrays by the specified value. This exampled is using the users variable created in the forEach section.
var sortedUsers = _.sortBy(users, 'first');
// Output
[{
age: 25,
email: "[email protected]",
first: "Cassandra",
fullName: "Cassandra Wilcox",
last: "Wilcox"
}, {
age: 25,
email: "[email protected]",
first: "Georgette",
fullName: "Georgette Keough",
last: "Keough"
}, {
age: 21,
email: "[email protected]",
first: "Ian",
fullName: "Ian Grail",
last: "Grail"
}, {
age: 35,
email: "[email protected]",
first: "Keith",
fullName: "Keith Keough",
last: "Keough"
}]
7) _.filter
Filter will allow you to return a new array of items based on logical criteria. This example again uses the users variable created in the forEach section.
var filteredByAge = _.filter(users, function(user) {
return user.age > 30;
});
// Output
[{
age: 35,
email: "[email protected]",
first: "Keith",
fullName: "Keith Keough",
last: "Keough"
}]
8) _.includes
This function will return a boolean value if your second argument is contained in the first argument (the array).
var names = ['keith', 'ian'];
_.includes(names, 'ian');
// Output
true
_.includes(names, 'georgette');
// Output
false
9) _.some
This lodash function is similar to _.includes, but allows you to work with arrays of objects. It will return true if your second argument is contained in the array. The nice thing about this function is you can match against the full object, or just part of it (on a specific field).
var users = [
{
first: 'Keith',
age: 35
},
{
first: 'Cassandra',
age: 25
},
{
first: 'Ian',
age: 21
},
{
first: 'Georgette',
age: 25
}
];
var user = {
first: 'Georgette',
age: 25
};
_.some(users, {first: 'Ian'});
// Output
true
_.some(users, {first: 'Billy'});
// Output
false
_.some(users, user);
// Output
true
I hope these handful of lodash features help you understand how powerful lodash can be for working with arrays, objects, and collections. You can head over to the docs to see many many more features that lodash has to offer.
About Author: Keith Keough
full stack dev, technology enthusiast